Adding custom fonts and colors to a Tailwind project
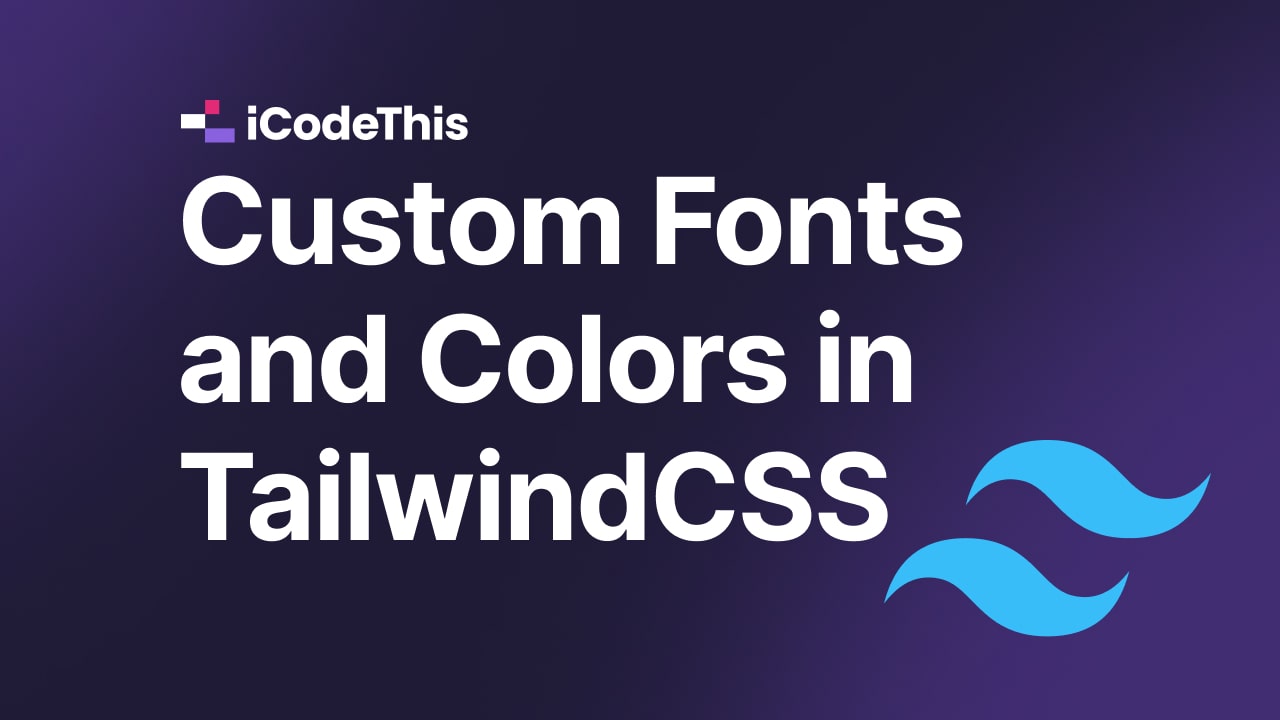
byMonimolimnionon Jul 24, 2023
Tailwind's CDN is a great way to test out the framework, and of course it can be used to rapidly produce great results on iCodeThis!
If you want to spice things up a little, you can easily use custom fonts and colors with the CDN version in a similar way to the installed version, using the Tailwind config object.
Adding the CDN
Every iCodeThis challenge starts with the Tailwind CDN already being imported, but if you've deleted it or want to add it a project of your own, it's simply a matter of addin the following script tag to your HTML head:
<script src="https://cdn.tailwindcss.com"></script>
Adding custom fonts
Adding a custom font is simple, using a service like Google Fonts. Find the font you want to use, add the appropriate weights to your selection, and copy the provided HTML link tags:
<link rel="preconnect" href="https://fonts.googleapis.com" />
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin />
<link
href="https://fonts.googleapis.com/css2?family=Nunito:wght@400;600&display=swap"
rel="stylesheet"
/>
Here I'm importing Nunito in 400 and 600 weights. Paste this into your HTML head:
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="preconnect" href="https://fonts.googleapis.com" />
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin />
<link
href="https://fonts.googleapis.com/css2?family=Nunito:wght@400;600&display=swap"
rel="stylesheet"
/>
<script src="https://cdn.tailwindcss.com"></script>
</head>
Now we need to tell Tailwind about our font so it can build it into the CSS. Open a new script tag and add a tailwind.config
object:
<script>
tailwind.config = {};
</script>
We want to alter the core theme
of Tailwind, so we add this object:
<script>
tailwind.config = {
theme: {},
};
</script>
We could start adding our custom fonts (and colors) inside this object, but that would override all the defaults. That might be desirable in our own projects, but it's often useful to have access to basic colours like black, white, and grey! So we can instead use the extend
object:
<script>
tailwind.config = {
theme: {
extend: {},
},
};
</script>
Now we can add our custom font using the fontFamily
object. We then have two options. We can override the default sans-serif font by assigning our custom font to the sans
property, specifying a fallback:
<script>
tailwind.config = {
theme: {
extend: {
fontFamily: {
sans: ["Nunito", "sans-serif"],
},
},
},
};
</script>
This will change all the sans-serif text in your project automatically. Or we can assign a custom font name:
<script>
tailwind.config = {
theme: {
extend: {
fontFamily: {
brand: ["Nunito", "sans-serif"],
},
},
},
};
</script>
We can then assign this font to any text element using font-brand
. Of course, if you import multiple fonts, you can add them as more entries in the fontFamily
object:
<script>
tailwind.config = {
theme: {
extend: {
fontFamily: {
title: ["Nunito", "sans-serif"],
body: ["Raleway", "sans-serif"],
},
},
},
};
</script>
Adding custom colours
Adding colours is just as easy, especially as we already have our extended theme set up. First add the colors
object inside extend
:
<script>
tailwind.config = {
theme: {
extend: {
fontFamily: {
brand: ["Nunito", "sans-serif"],
},
colors: {},
},
},
};
</script>
For simple, one off colours, you can simply give a key value pair of name:value. You can use any CSS color format :
<script>
tailwind.config = {
theme: {
extend: {
fontFamily: {
brand: ["Nunito", "sans-serif"],
},
colors: {
"brand-blue": "#04c2c1",
"brand-red": "hsl(9,58%,52%)",
},
},
},
};
</script>
Or if you want to use variations of a colour, you can use a further nested object:
<script>
tailwind.config = {
theme: {
extend: {
fontFamily: {
brand: ["Nunito", "sans-serif"],
},
colors: {
"brand-blue": {
DEFAULT: "#04c2c1",
light: "#2bf0f0",
dark: "#054f4f",
},
"brand-red": "hsl(9,58%,52%)",
},
},
},
};
</script>
This will generate color classes such as text-brand-blue
, text-brand-blue-light
, and text-brand-blue-dark
for all the Tailwind utilities that use colour, such as bg-
, shadow-
, border-
and so on.
And that's it! Using these tips, you can put the finishing touches on your iCodeThis challenges, and customise your own projects - the same approach of extending the theme
object applies to the installed version of Tailwind, where you would use a tailwind.config.js
file.